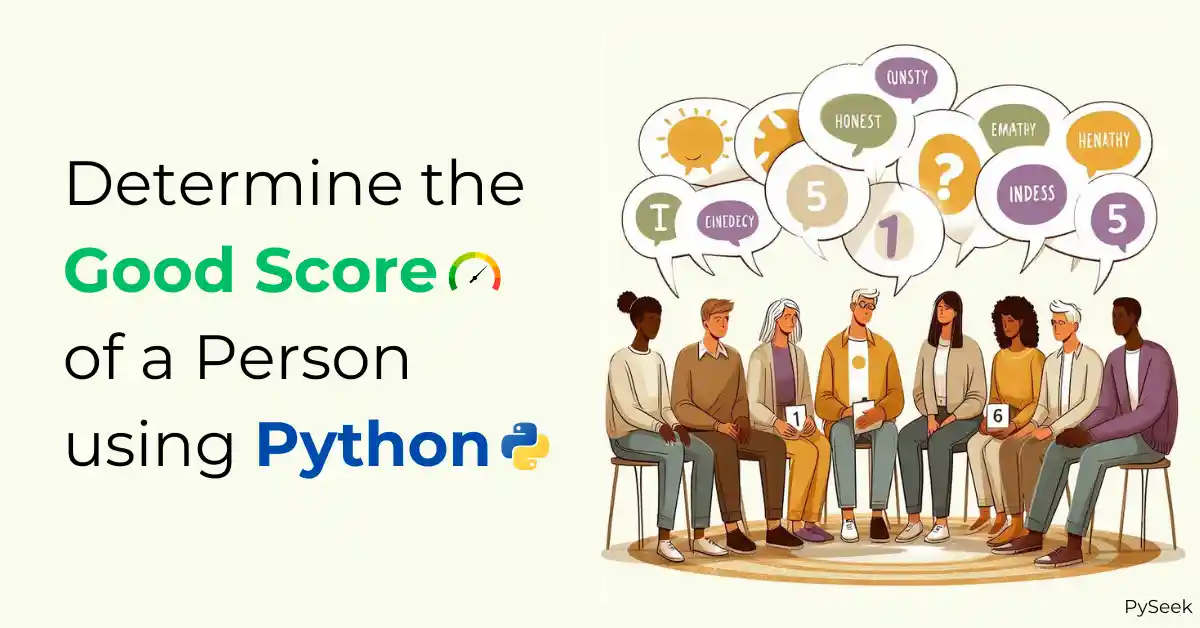
Introduction
Is there such a thing as a âgoodâ or âbadâ person? Itâs a question that philosophers have pondered for centuries. Human nature is a complex tapestry woven with countless threads of experience, upbringing, and circumstance. Trying to label someone as inherently good or bad based on a few simple metrics is like trying to judge a book by its cover.
However, in the realm of programming, we can create a simplified model to assess different dimensions of a personâs character. In this article, weâll explore how to develop a Python program that calculates a âgood scoreâ for a person based on a series of questions.
This program asks the user 24 questions that cover different aspects of personality, values, and behavior. By answering these questions on a scale from 1 to 5, the program calculates an average score and gives feedback based on that score.
How to Determine the Good Score of a Person
To evaluate a personâs âgood score,â we focus on key character traits that are generally associated with positive behavior. Below are 12 categories, each with two questions that help measure different dimensions of goodness:
1. Honesty
- How often do you tell the truth, even if it could lead to consequences for you? (1-5)
- How often do you lie to get out of a difficult situation? (1-5)
2. Empathy
- How often do you try to understand and help others who are going through a tough time? (1-5)
- When someone is hurt, how often do you feel the need to assist them? (1-5)
3. Responsibility
- How often do you fulfill your commitments, even when itâs difficult? (1-5)
- How often do you take responsibility for your mistakes rather than blaming others? (1-5)
4. Respect for Others
- How often do you treat others with kindness and respect, regardless of their background? (1-5)
- How often do you intentionally disrespect someone for personal gain? (1-5)
5. Self-Control
- How often can you control your temper, even in stressful situations? (1-5)
- How often do you avoid temptations or harmful habits? (1-5)
6. Generosity
- How often do you share your time, resources, or skills with others? (1-5)
- How often do you refuse to help someone in need when you have the ability to do so? (1-5)
7. Forgiveness
- How often are you quick to forgive someone who wronged you? (1-5)
- How often do you hold grudges for a long time when someone hurts you? (1-5)
8. Humility
- How often do you recognize your own flaws and weaknesses? (1-5)
- How often do you act arrogantly, thinking you are better than others? (1-5)
9. Fairness
- How often do you treat everyone fairly, even when itâs difficult or inconvenient for you? (1-5)
- How often do you take advantage of someone because it was easy? (1-5)
10. Courage
- How often do you stand up for what is right, even if you are afraid of the consequences? (1-5)
- How often do you remain silent when you see something wrong happening? (1-5)
11. Work Ethic
- How often do you give your best effort in tasks, even when no one is watching? (1-5)
- How often do you avoid work or responsibilities that you should have done? (1-5)
12. Open-Mindedness
- How often are you open to considering perspectives and ideas different from your own? (1-5)
- How often do you dismiss someoneâs opinion without considering their point of view? (1-5)
By answering these questions on a scale of 1 to 5 (1 being ârarelyâ and 5 being âalwaysâ), we can determine a personâs overall good score. The program calculates the average of all answers, providing a simple metric for goodness.
The Source Code
Now that weâve identified the key categories and questions, letâs take a look at the Python program that will ask these questions, calculate the total score, and provide feedback based on the average score.
def calculate_good_score(): """ Calculates a simplified "goodness" score based on user input (1-5 scale). """ # Define questions questions = [ # Honesty "How often do you tell the truth, even if it could lead to consequences for you? (1-5)", "How often do you lie to get out of a difficult situation? (1-5)", # Empathy "How often do you try to understand and help others who are going through a tough time? (1-5)", "When someone is hurt, how often do you feel the need to assist them? (1-5)", # Responsibility "How often do you fulfill your commitments, even when it's difficult? (1-5)", "How often do you take responsibility for your mistakes rather than blaming others? (1-5)", # Respect for Others "How often do you treat others with kindness and respect, regardless of their background? (1-5)", "How often do you intentionally disrespect someone for personal gain? (1-5)", # Self-Control "How often can you control your temper even in stressful situations? (1-5)", "How often do you avoid temptations or harmful habits? (1-5)", # Generosity "How often do you share your time, resources, or skills with others? (1-5)", "How often do you refuse to help someone in need when you have the ability to do so? (1-5)", # Forgiveness "How often are you quick to forgive someone who wronged you? (1-5)", "How often do you hold grudges for a long time when someone hurts you? (1-5)", # Humility "How often do you recognize your own flaws and weaknesses? (1-5)", "How often do you act arrogantly, thinking you are better than others? (1-5)", # Fairness "How often do you treat everyone fairly, even when it's difficult or inconvenient for you? (1-5)", "How often do you take advantage of someone because it was easy? (1-5)", # Courage "How often do you stand up for what is right, even if you are afraid of the consequences? (1-5)", "How often do you remain silent when you see something wrong happening? (1-5)", # Work Ethic "How often do you give your best effort in tasks, even when no one is watching? (1-5)", "How often do you avoid work or responsibilities that you should have done? (1-5)", # Open-Mindedness "How often are you open to considering perspectives and ideas different from your own? (1-5)", "How often do you dismiss someone's opinion without considering their point of view? (1-5)" ] total_score = 0 for question in questions: while True: try: answer = int(input(question)) if 1 <= answer <= 5: total_score += answer break else: print("Please enter a number between 1 and 5.") except ValueError: print("Invalid input. Please enter a number between 1 and 5.") # Calculate average score average_score = total_score / len(questions) # Determine personality based on a simplified scale if average_score >= 4: personality = "You are a generally good person with strong values." elif average_score >= 3: personality = "You have a good foundation but could improve in some areas." else: personality = "You might want to focus on developing empathy and integrity." print(f"\nYour average score is: {average_score:.2f}") print(personality) if __name__ == "__main__": calculate_good_score()
Output
The program will ask you a series of 24 questions. Answer each question by entering a value between 1 and 5 (1 being ârarelyâ and 5 being âalwaysâ).
How often do you tell the truth, even if it could lead to consequences for you? (1-5): 3 How often do you lie to get out of a difficult situation? (1-5): 5 How often do you try to understand and help others who are going through a tough time? (1-5): 4 When someone is hurt, how often do you feel the need to assist them? (1-5): 4 How often do you fulfill your commitments, even when it's difficult? (1-5): 4 How often do you take responsibility for your mistakes rather than blaming others? (1-5): 4 How often do you treat others with kindness and respect, regardless of their background? (1-5): 3 How often do you intentionally disrespect someone for personal gain? (1-5): 1 How often can you control your temper even in stressful situations? (1-5): 3 How often do you avoid temptations or harmful habits? (1-5): 3 How often do you share your time, resources, or skills with others? (1-5): 4 How often do you refuse to help someone in need when you have the ability to do so? (1-5): 2 How often are you quick to forgive someone who wronged you? (1-5): 4 How often do you hold grudges for a long time when someone hurts you? (1-5): 2 How often do you recognize your own flaws and weaknesses? (1-5): 4 How often do you act arrogantly, thinking you are better than others? (1-5): 1 How often do you treat everyone fairly, even when it's difficult or inconvenient for you? (1-5): 4 How often do you take advantage of someone because it was easy? (1-5): 3 How often do you stand up for what is right, even if you are afraid of the consequences? (1-5): 2 How often do you remain silent when you see something wrong happening? (1-5): 3 How often do you give your best effort in tasks, even when no one is watching? (1-5): 4 How often do you avoid work or responsibilities that you should have done? (1-5): 2 How often are you open to considering perspectives and ideas different from your own? (1-5): 5 How often do you dismiss someone's opinion without considering their point of view? (1-5): 2 Your average score is: 3.17 You have a good foundation but could improve in some areas.
Summary
In this article, we developed a Python program that determines the good score of a personâs character by asking thoughtful questions across various categories such as honesty, empathy, responsibility, and more.
Youâll be asked to rate your behavior on a scale from 1 to 5, allowing the program to calculate an average score that reflects your overall âgoodness.â Remember, this isnât a definitive judgment of your character, but rather a reflective exercise to encourage self-awareness and personal growth.
Whether youâre seeking a fun diversion or a tool for self-improvement, this program can be a valuable companion on your journey toward understanding who you are and the values that shape your actions.
For more examples like this, visit our dedicated page Python Programming Examples. Here are a few examples to spark your interest:
- Wish Happy Birthday using Python Programs
- Say I Love You using a Python Code
- Creating a Colorful Sketch of Messi using a Python Code
- Creating a Matrix Effect in Python with Curses
- Learn How to Encrypt a Text Message in Python
Happy Coding!