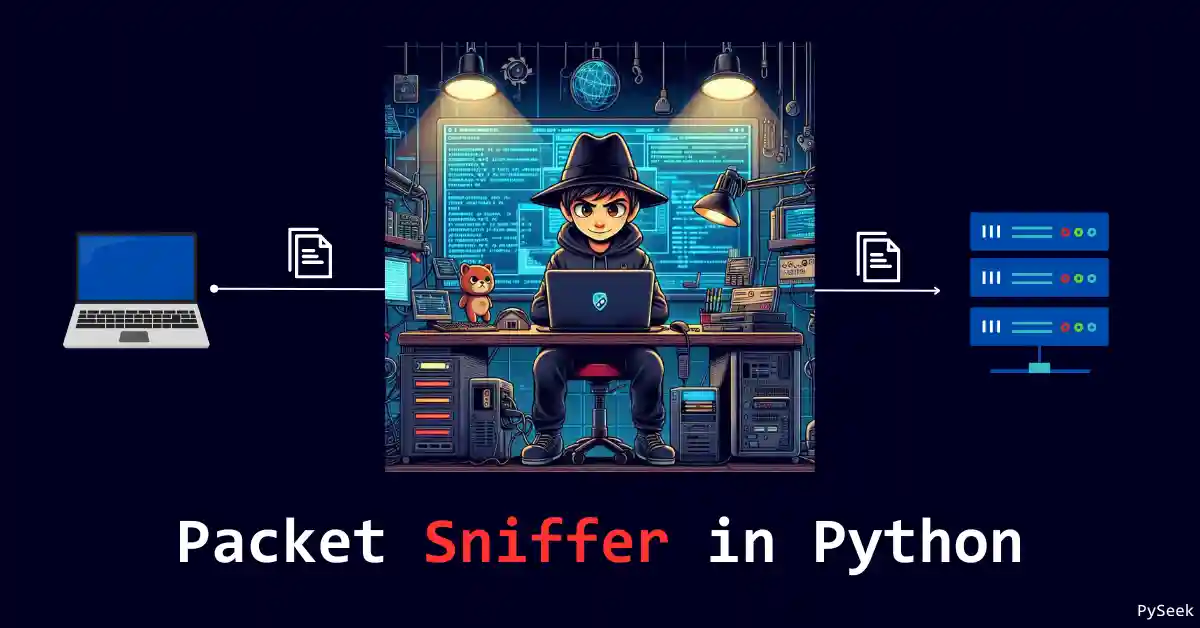
Introduction
In this tutorial, we will explore how to create a simple packet sniffer and analyzer using Python. A packet sniffer is a tool used to capture and analyze network traffic. This can be useful for network diagnostics, monitoring, and educational purposes. We will use the Scapy library in Python, which makes it easy to handle network packets.
Disclaimer
This tutorial is intended for educational purposes only. The techniques demonstrated here should only be used on networks where you have explicit permission to monitor the traffic. Unauthorized use of packet sniffing tools can be illegal and unethical.
Visit Also: Create a Python Network Scanner: Find IPs & MACs
Requirements and Installations
Before we begin, make sure Python is installed on your system. You can download it from python.org. Next, you need Scapy, a powerful Python library used for network packet manipulation. Install it using the following command:
pip install scapy
The Program
Choose your favorite text editor or IDE and create a Python file named āpacket_sniffer.pyā. Now copy the following code into that file and save it.
from scapy.all import sniff from scapy.layers.inet import IP, TCP, UDP, ICMP def packet_callback(packet): if IP in packet: ip_layer = packet[IP] protocol = ip_layer.proto src_ip = ip_layer.src dst_ip = ip_layer.dst # Determine the protocol protocol_name = "" if protocol == 1: protocol_name = "ICMP" elif protocol == 6: protocol_name = "TCP" elif protocol == 17: protocol_name = "UDP" else: protocol_name = "Unknown Protocol" # Print packet details print(f"Protocol: {protocol_name}") print(f"Source IP: {src_ip}") print(f"Destination IP: {dst_ip}") print("-" * 50) def main(): # Capture packets on the default network interface sniff(prn=packet_callback, filter="ip", store=0) if __name__ == "__main__": main()
Explanation
- packet_callback Function:
- This function processes each captured packet.
- It checks if the packet has an IP layer.
- It extracts the protocol number, source IP, and destination IP from the IP layer.
- It determines the protocol name based on the protocol number.
- It prints the protocol name, source IP, and destination IP.
- main Function:
- This function starts capturing packets on the default network interface.
- The āsniffā function is called with āprn=packet_callbackā to specify the callback function for each captured packet, filter=āipā to capture only IP packets, and āstore=0ā to avoid storing packets in memory.
How to Test the Program
Please follow the steps below to check the program is working.
Run the Program
Open a terminal or command prompt, navigate to the directory where you saved the program, and run it with the following command:
sudo python packet_sniffer.py
Note: On Windows, you might not need āsudoā (you might need to run the program with administrator privileges), but on Unix-like systems (Linux, macOS), you usually need āsudoā to run the program with the necessary permissions to capture network packets.
Generate Network Traffic
- Open a web browser and visit a few websites.
- Use other network applications like email clients or chat applications.
- Use command-line tools like āpingā or ācurlā to generate additional traffic.
Output
The output should look something like this:
Protocol: TCP Source IP: 192.168.1.2 Destination IP: 93.184.216.34 -------------------------------------------------- Protocol: TCP Source IP: 192.168.1.2 Destination IP: 172.217.14.206 -------------------------------------------------- Protocol: UDP Source IP: 192.168.1.2 Destination IP: 8.8.8.8 --------------------------------------------------
Visit Also: Create a Simple Brute-Force Password Cracker in Python
Summary
In this tutorial, we have created a basic packet sniffer and analyzer using Python and the Scapy library. This tool captures network traffic and prints the protocol, source IP, and destination IP of each packet.
Remember to use this tool ethically and only on networks where you have permission. This educational tutorial aims to help in understanding network protocols and packet analysis.
For any queries, reach out to me at contact@pyseek.com.
Happy Coding!