Python Tutorials
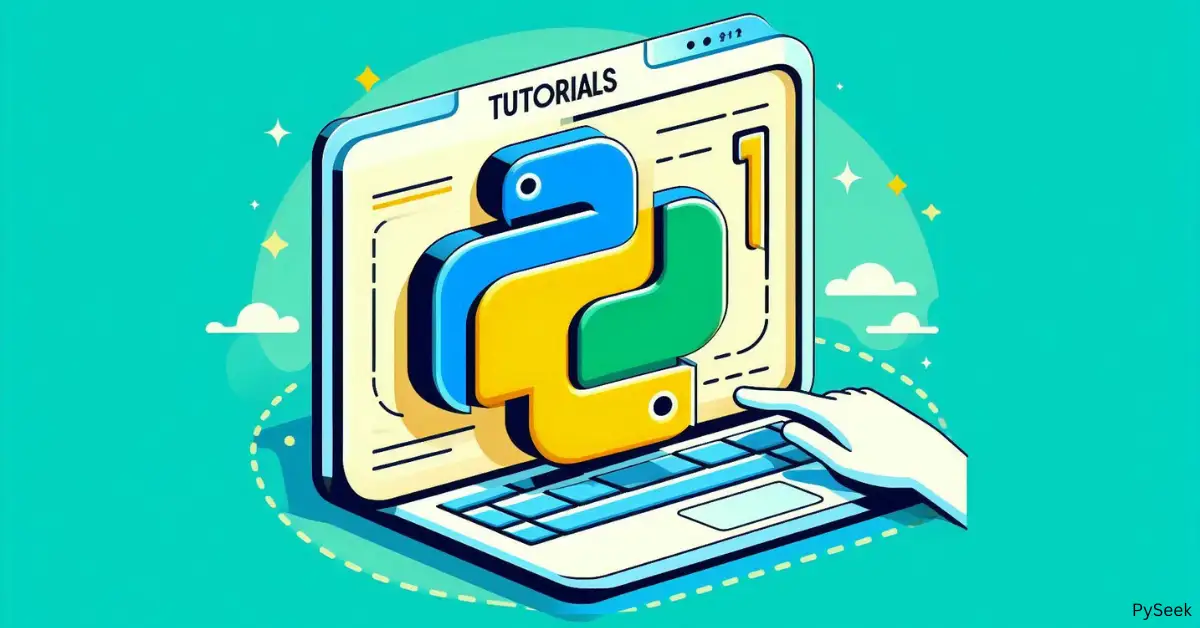
Basic Tutorials
Introduction The print function in Python is a built-in function that allows you to display messages...
Introduction In Python, the input function is a handy tool for getting information from the user. It...
Introduction In computer programming, variables are used to store information that can be used...
Introduction Strings are an essential data type in Python. A string is a sequence of characters that...
Conditional statements, or decision-making statements, are fundamental building blocks in...
Introduction In Python, a list is a fundamental data structure that allows you to store an ordered...
Introduction Sorting a Python List means arranging all the elements in a certain order. The order...
Introduction Python is a widely used programming language that comes with a range of built-in data...
Introduction A tuple in Python is an ordered collection of values that can be of different data...
Introduction Python sets are an incredibly useful data type in the Python programming language. A...
Introduction File handling is a crucial aspect of programming that allows you to read and write data...
Introduction List comprehension is a concise and powerful tool for creating lists in Python. It...
Introduction Dictionary comprehension is a powerful feature in Python that allows you to create...
In Python, a list of lists is a powerful tool for handling multi-dimensional data. It’s...
Advanced Tutorials
Introduction PDF (Portable Document Format) files are widely used for sharing and presenting...
Introduction Regular expressions are powerful tools used for pattern matching and manipulation of...
Introduction Python, being a dynamically typed language, offers various tools and features to...
Introduction CSV (Comma-Separated Values) files are a widely used format for storing tabular data...
Introduction In the realm of programming, performance is a key factor that often determines the...
Introduction Object-Oriented programming (OOP) is the most popular programming paradigm based on the...
Introduction Command-line arguments allow users to pass information to a Python script when it is...
Introduction In Python, lambda functions are anonymous functions that allow you to create a small...
Introduction Python decorators are a powerful feature of the language that allows you to modify the...
Introduction Excel is a widely used tool for data analysis, but sometimes it can be difficult to...
Introduction In the realm of file and directory manipulation, Python offers a versatile module...
Introduction In Python, working with dates and times is a common task in many applications. Whether...
Introduction Python is a versatile and powerful programming language that has gained widespread...
Introduction Python is a versatile and widely-used programming language known for its simplicity and...
Introduction If you’re diving into the world of Python programming, you’ve likely come...
Python is known for its simplicity and flexibility, which is why it’s a popular programming...
In automation and task management, scheduling plays a crucial role. Python offers several tools and...
Testing is a crucial part of any software development process. It helps ensure that your code works...