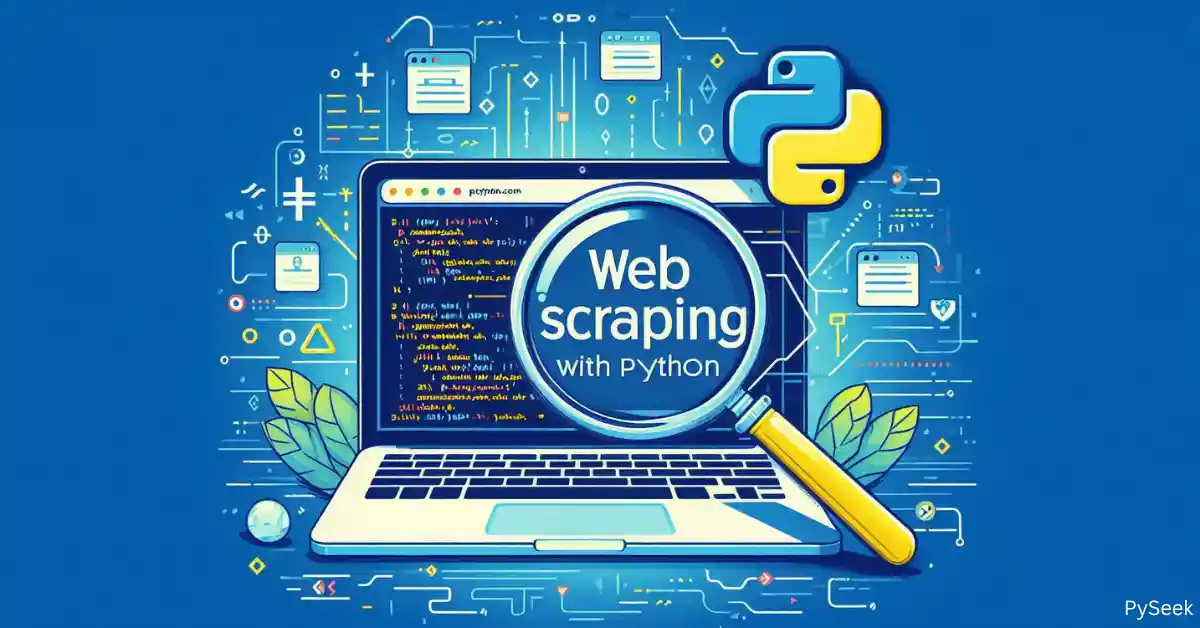
Web scraping is a powerful technique that allows you to extract data from websites automatically. Whether youāre gathering information for research, analyzing competitors, or building a dataset for a machine learning project, web scraping can save you hours of manual work. In this tutorial, weāll walk you through how to scrape websites using two popular Python libraries: BeautifulSoup and Requests.
By the end of this article, youāll have a solid understanding of how to scrape websites efficiently and ethically. Letās dive in!
Read Also: Create a YouTube Downloader in Python using Tkinter
What is Web Scraping?
Web scraping is the process of extracting data from websites. It involves sending a request to a website, downloading its HTML content, and then parsing that content to extract the information you need. While some websites offer APIs to access their data, many do not, making web scraping a valuable skill.
Why Use BeautifulSoup and Requests?
- Requests: This library is used to send HTTP requests in Python. It allows you to fetch the HTML content of a webpage effortlessly.
- BeautifulSoup: This library is used to parse HTML and XML documents. It creates a parse tree that makes it easy to extract data from the HTML structure.
Together, these libraries make web scraping simple and efficient, even for beginners.
Requirements
Before we start, make sure you have Python installed on your system. Youāll also need to install the required libraries. You can do this using pip:
pip install requests beautifulsoup4
Step 1: Inspect the Website
Before writing any code, you need to understand the structure of the website you want to scrape. Hereās how:
- Open the website in your browser.
- Right-click on the page and select Inspect (or press Ctrl+Shift+I on Windows or Cmd+Option+I on Mac).
- Use the Elements tab to explore the HTML structure. Pay attention to the tags, classes, and IDs of the elements you want to scrape.
For example, if you want to scrape product names from an e-commerce site, look for the HTML tags that contain the product names.
Step 2: Send a Request to the Website
Once youāve identified the data you want to scrape, the next step is to fetch the HTML content of the webpage. This is where the Requests library comes in.
Hereās an example:
import requests # URL of the website you want to scrape url = "https://example.com" # Send a GET request to the website response = requests.get(url) # Check if the request was successful if response.status_code == 200: print("Request successful!") html_content = response.text # Get the HTML content else: print(f"Failed to retrieve the webpage. Status code: {response.status_code}")
Step 3: Parse the HTML Content
Now that you have the HTML content, you need to parse it to extract the data. This is where BeautifulSoup shines.
Hereās how to use it:
from bs4 import BeautifulSoup # Parse the HTML content using BeautifulSoup soup = BeautifulSoup(html_content, "html.parser") # Example: Extract the title of the webpage title = soup.title.string print(f"Title of the webpage: {title}")
Step 4: Extract Data from the HTML
Once the HTML is parsed, you can use BeautifulSoupās methods to extract specific elements. Here are some common techniques:
Extracting Text from Tags
# Extract all paragraphs paragraphs = soup.find_all("p") for p in paragraphs: print(p.text)
Extracting Elements by Class or ID
# Extract elements with a specific class products = soup.find_all("div", class_="product-name") for product in products: print(product.text) # Extract an element by ID header = soup.find(id="header") print(header.text)
Extracting Links
# Extract all links on the page links = soup.find_all("a") for link in links: print(link.get("href"))
Step 5: Handle Pagination or Multiple Pages
Many websites spread data across multiple pages. To scrape all the data, youāll need to handle pagination. Hereās an example:
base_url = "https://example.com/products?page=" for page in range(1, 6): # Scrape the first 5 pages url = base_url + str(page) response = requests.get(url) soup = BeautifulSoup(response.text, "html.parser") # Extract data from each page products = soup.find_all("div", class_="product") for product in products: print(product.text)
Step 6: Save the Scraped Data
Once youāve extracted the data, youāll likely want to save it for further analysis. You can save it in various formats, such as CSV, JSON, or a database.
Hereās an example of saving data to a CSV file:
import csv # Data to save data = [["Product Name", "Price"], ["Product 1", "$10"], ["Product 2", "$20"]] # Save to CSV with open("products.csv", "w", newline="") as file: writer = csv.writer(file) writer.writerows(data)
Ethical Web Scraping: Best Practices
While web scraping is a powerful tool, itās important to use it responsibly. Here are some best practices:
- Respect Robots.txt: Check the websiteās robots.txt file to see if scraping is allowed.
- Limit Request Rate: Avoid sending too many requests in a short period. Use time.sleep() to add delays between requests.
- Identify Yourself: Some websites may block requests that donāt have a user-agent header. Add a user-agent to your requests to identify yourself.
- Donāt Scrape Sensitive Data: Avoid scraping personal or sensitive information.
Common Challenges and Solutions
- Dynamic Content: Some websites load content dynamically using JavaScript. In such cases, you may need to use a tool like Selenium.
- IP Blocking: Websites may block your IP if they detect unusual activity. Use proxies to avoid this.
- Changing HTML Structure: Websites often update their HTML, which can break your scraper. Regularly check and update your code.
Conclusion
Web scraping with BeautifulSoup and Requests is a straightforward and effective way to extract data from websites. By following the steps outlined in this guide, you can scrape data efficiently while adhering to ethical practices.
Remember, web scraping is a skill that improves with practice. Start with simple projects, and gradually tackle more complex websites.
If you found this guide helpful, feel free to share it with others who might benefit from it. And if you have any questions or run into issues, reach out to me at contact@pyseek.com.
Happy scraping!