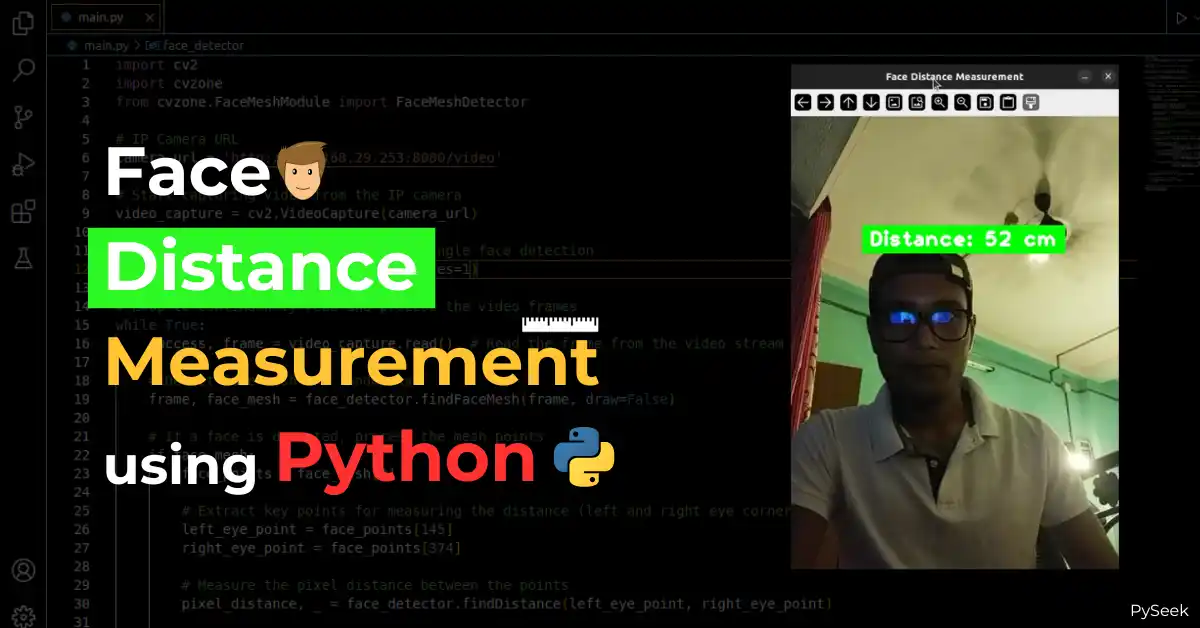
Introduction
Measuring the distance between your face and a computer screen in real time can be a valuable tool, especially for health-conscious individuals and ergonomic setups. In this project, we will demonstrate how to achieve this using Python libraries such as OpenCV and cvzone. The main objective is to perform real-time face distance measurement from the screen using a simple webcam. This technique is based on facial landmarks and mathematical calculations.
The project will use the āFaceMeshDetectorā from the ācvzoneā library to detect facial landmarks and measure the distance between the face and the screen. The distance calculation relies on known measurements and the focal length of the camera, which weāll extract during the process.
By the end of this tutorial, you will have a program that continuously monitors your face distance from the screen, displaying it in centimeters. Letās dive into the details of how to achieve this.
How to Measure the Distance Between Computer Screen and Face
To measure the distance between your face and the screen, we need to understand some basic concepts of image processing and mathematics. Hereās how it works:
- Facial Landmarks: Using the āFaceMeshDetectorā, we detect specific points on the face, such as the eyeball of the eyes. These points help us determine the distance in pixels between them (letās call this āpixel_distanceā).
- Real-World Width: This is the actual distance between the two points on your face, such as the distance between the corners of your eyes, in centimeters. In this project, weāll assume this width is 6.3 cm.
- Focal Length Calculation: The focal length of the camera can be determined using the formula:
focal_length = (pixel_distance * distance_to_face)/real_world_width
. For this project, we extract the focal length by measuring the pixel distance and the actual distance of the face from the camera. - Real-Time Distance Measurement: Once the focal length is known, you can calculate the distance between your face and the screen in real-time using the formula:
distance_to_face = (real_world_width * focal_length) / pixel_distance
.
This mathematical approach allows the program to calculate the distance dynamically as your face moves closer to or further away from the screen.
Requirements and Installation
To run this project, you need the following:
Python 3.x: Ensure you have Python installed on your system. You can download it from their official site www.python.org/downloads.
OpenCV: Install it using the following command (Documentation):
pip install opencv-python
cvzone: Install it using the following command (Documentation):
pip install cvzone
The Source Code
Hereās the source code for the real-time face distance measurement project, broken down into several parts for easier understanding:
Setting Up the Video Stream and Face Detection
import cv2 import cvzone from cvzone.FaceMeshModule import FaceMeshDetector # Start capturing video from the WebCam video_capture = cv2.VideoCapture(0) # Initialize FaceMeshDetector with a single face detection face_detector = FaceMeshDetector(maxFaces=1)
In this part, we import the required libraries and set up the video stream from a webcam. We also initialize the FaceMeshDetector to detect one face.
Processing the Video Stream and Extracting Facial Landmarks
while True: success, frame = video_capture.read() # Read the frame from the video stream # Detect face mesh and landmarks frame, face_mesh = face_detector.findFaceMesh(frame, draw=False)
Here, we start reading frames from the video stream and detect facial landmarks using the FaceMeshDetector. The draw=False
option avoids drawing the landmarks directly on the frame.
Measuring the Distance Between Eye Balls
# If a face is detected, process the mesh points if face_mesh: face_points = face_mesh[0] # Extract key points for measuring the distance (left and right eye balls) left_eye_point = face_points[145] right_eye_point = face_points[374]
In this section, we extract the specific points on the face (left and right eye balls) and draw a line between them to visualize the measurement.
Calculating Distance
# Measure the pixel distance between the points pixel_distance, _ = face_detector.findDistance(left_eye_point, right_eye_point) # Real-world width of the object (distance between the eyes in cm) real_world_width = 6.3 # Comment out the below lines to calculate the focal length # focal_length = (pixel_distance * distance_to_face)/real_world_width # print(focal_length) # Focal length (previously calculated) focal_length = 530 # Calculate the approximate distance from the camera to the face distance_to_face = (real_world_width * focal_length) / pixel_distance if distance_to_face < 50: cvzone.putTextRect(frame, f'Distance: {int(distance_to_face)} cm', (face_points[10][0] - 75, face_points[10][1] - 50), scale=2, colorR=(0, 0, 255), font=cv2.FONT_HERSHEY_PLAIN) elif 50 <= distance_to_face <= 76: # Display the calculated distance on the frame cvzone.putTextRect(frame, f'Distance: {int(distance_to_face)} cm', (face_points[10][0] - 75, face_points[10][1] - 50), scale=2, colorR=(0, 255, 0), font=cv2.FONT_HERSHEY_PLAIN) else: cvzone.putTextRect(frame, f'Distance: {int(distance_to_face)} cm', (face_points[10][0] - 75, face_points[10][1] - 50), scale=2, colorR=(255, 0, 0), font=cv2.FONT_HERSHEY_PLAIN)
Here, we calculate the real-time distance between the face and the screen using the formulas discussed earlier. The result is displayed in centimeters on the frame.
Displaying the Output and Handling Exit
# Show the processed video frame cv2.imshow("Face Distance Measurement", frame) # Exit loop if 'q' is pressed if cv2.waitKey(1) & 0xFF == ord('q'): break # Release the video capture and close any OpenCV windows video_capture.release() cv2.destroyAllWindows()
This final part handles displaying the output and exits the loop when the user presses the āqā key.
The Output
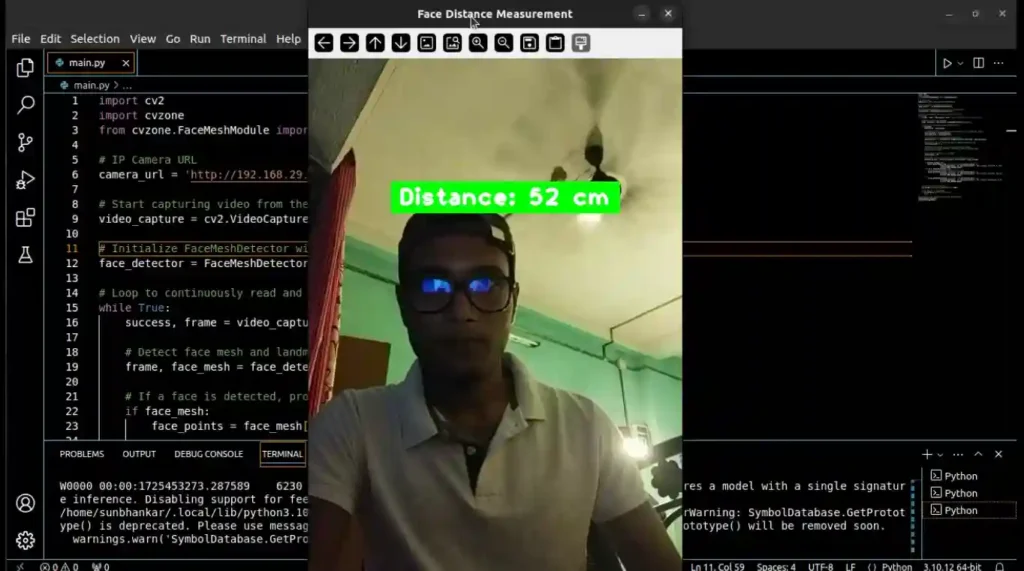
Summary
In this project, we explored how to perform real-time face distance measurement from a computer screen using Python, OpenCV, and cvzone. By using facial landmarks and simple mathematical formulas, we were able to calculate the distance between your face and the screen in real-time.
This technique can be useful for ergonomic purposes and maintaining a healthy distance from your screen. We also provided a breakdown of the code for easy understanding, especially for beginners interested in computer vision.
For any query related to this project, reach out to me at contact@pyseek.com.
Explore more interesting topics here: Python Projects. Below are a few examples to spark your interest:
- Real-time Glass Detection on Face using Python
- Create a Face Filter in Python: Lips Color Changing Project
- Create an Image to Pencil Sketch Converter in Python
- Create an Image to Cartoon Converter using Python OpenCV
Happy Measuring!